'+
'
'+
'
'+
'
'+
'
'+ (LANG.hasOwnProperty(LID) ? getText("labels", "uni") : default_title) + '
'+
'
'+
'
'+
'
'+
'
'+ ITowns.town_groups._byId[groupArray[-1].id].attributes.name +'
'+
'
'+
'
'+
'
'+
'
'+
'
'+
'
').appendTo('body');
for(var group in groupArray){
if(groupArray.hasOwnProperty(group)){
var group_name = ITowns.town_groups._byId[group].attributes.name;
$('
'+ group_name +'
').appendTo('#available_units_box .item-list');
}
}
// Styles
$('#available_units_box .drop_box').css({
float: 'left',
position: 'absolute',
top: '1px',
right: '0px',
width: '90px',
zIndex: '1'
});
$('#available_units_box h4').css({
color: 'rgb(128, 64, 0)',
width: '10px',
height: '25px',
marginLeft: '4px',
lineHeight: '1.9'
});
$('#available_units_box .drop_group').css({
width: '84px'
});
$('#available_units_box .select_group').css({
position: 'absolute',
width: '80px',
display: "none",
right: '3px'
});
//$('#available_units_box .item-list').css({ maxHeight: '400px', maxWidth: '200px', align: "right" });
$('#available_units_box .arrow').css({
width: '18px',
height: '18px',
background: 'url('+ drop_out.src +') no-repeat -1px -1px',
position: 'absolute'
});
// hover effects of the elements in the drop menu
$('#available_units_box .option').hover(
function(){ $(this).css({color: '#fff', background: "#328BF1"}); },
function(){ $(this).css({color: '#000', background: "#FFEEC7"}); }
);
// click events of the drop menu
$('#available_units_box .select_group .option').each(function(){
$(this).click(function(e){
$(this).parent().find(".sel").toggleClass("sel");
$(this).toggleClass("sel");
$('#available_units_box .drop_group .caption').attr("name", $(this).attr("name"));
$('#available_units_box .drop_group .caption').get(0).innerHTML = $(this).get(0).innerHTML;
$('#available_units_box .select_group')[0].style.display = "none";
updateAvailableUnitsBox(groupUnitArray[$(this).attr("name")]);
//$('#available_units_box .drop_group .caption').change();
});
});
// show & hide drop menu on click
$('#available_units_box .drop_group').click(function(){
if($('#available_units_box .select_group')[0].style.display === "none"){
$('#available_units_box .select_group')[0].style.display = "block";
} else {
$('#available_units_box .select_group')[0].style.display = "none";
}
});
$('#available_units_box').click(function(e){
var clicked = $(e.target);
if(!(clicked[0].parentNode.className.split(" ")[1] === "dropdown")){
$('#available_units_box .select_group').get(0).style.display = "none";
}
});
// hover arrow change
$('#available_units_box .dropdown').hover(function(e){
$(e.target)[0].parentNode.childNodes[3].style.background = "url('"+ drop_over.src +"') no-repeat -1px -1px";
}, function(e){
$(e.target)[0].parentNode.childNodes[3].style.background = "url('"+ drop_out.src +"') no-repeat -1px -1px";
});
//$("#available_units_box .drop_group .caption").attr("name", "All");
//$('#available_units_box .drop_group').tooltip();
$('#available_units_box').draggable({
containment: "body",
snap: "body",
});
$('#available_units_box').css({
color: 'rgb(12, 69, 12)',
position: 'absolute',
top: '100px',
left: '200px',
zIndex: getMaxZIndex() + 1,
display: 'none'
});
$('#available_units_box .box_content').css({
background: 'url(http://s1.directupload.net/images/140206/8jd9d3ec.png) 94% 94% no-repeat',
backgroundSize: '140px'
});
$('#available_units_box').bind("mousedown",function(){
$(this).get(0).style.zIndex = getMaxZIndex() + 1;
});
$('#available_units_box hr').css({ margin: '3px 0px 0px', border: '1px solid', color: 'rgb(128, 64, 0)'});
} catch(error){
errorHandling(error, "addAvailableUnitsBox");
}
}
function updateAvailableUnitsBox(unitArray){
var i = 0, content = '
', ttpArray = {};
for(var u in unitArray){
if(unitArray.hasOwnProperty(u)){
if(((i%5 == 0) && (i!== 25)) || u == "bireme") {
content += " |
";
}
content += ' ' + unitArray[u] + ' ';
ttpArray[u] = GameData.units[u].name;
i++;
}
}
content += ' |
';
$('#available_units_box .box_content').get(0).innerHTML = "";
$('#available_units_box .box_content').append(content);
// Unit name tooltips
for(var o in ttpArray){
if(ttpArray.hasOwnProperty(o)){
$("#available_units_box ."+ o).tooltip(ttpArray[o]);
}
}
}
function unbindFavorPopup(){
//$('.gods_favor_button_area, #favor_circular_progress').mouseover();
//$('.gods_favor_button_area, #favor_circular_progress').mouseout();
$('.gods_favor_button_area, #favor_circular_progress').bind('mouseover mouseout', function(){
return false;
});
$('.gods_area').bind('mouseover', function(){
setFavorPopup();
});
}
var godArray = {
zeus: ' 0px', //'http://s1.directupload.net/images/140116/mkhzwush.png',
hera: '-152px', //'http://s1.directupload.net/images/140116/58ob8z82.png',
poseidon: '-101px', //'http://s1.directupload.net/images/140116/dkfxrw2f.png',
athena: ' -50px', //'http://s14.directupload.net/images/140116/iprgopak.png',
hades: '-203px', //'http://s14.directupload.net/images/140116/c9juk95y.png',
artemis: '-305px', //'http://s14.directupload.net/images/140116/pdc8vxe2.png'
};
var godImg = new Image(); godImg.src = "http://diotools.pf-control.de/images/game/gods.png";
function setFavorPopup(){
var pic_row = "",
fav_row = "",
prod_row = "";
for(var g in godArray){
if(godArray.hasOwnProperty(g)){
if(uw.ITowns.player_gods.attributes.temples_for_gods[g]){
pic_row += '
| ';
fav_row += '
'+ uw.ITowns.player_gods.attributes[g + "_favor"] +' | ';
prod_row += '
'+ uw.ITowns.player_gods.attributes.production_overview[g].production +' | ';
}
}
}
var tool_element = $('
| '+ pic_row +'
'+
' | '+ fav_row +'
'+
'+ | '+ prod_row +'
'+
'
');
$('.gods_favor_button_area, #favor_circular_progress').tooltip(tool_element);
}
/*******************************************************************************************************************************
* GUI Optimization
* ----------------------------------------------------------------------------------------------------------------------------
* | ● Modified spell box (smaller, moveable & position memory)
* | ● Larger taskbar and minimize daily reward-window on startup
* | ● Modify chat
* | ● Improved display of troops and trade activity boxes (movable with position memory on startup)
* ----------------------------------------------------------------------------------------------------------------------------
*******************************************************************************************************************************/
// Spell box
function catchSpellBox(){
$.Observer(uw.GameEvents.ui.layout_gods_spells.rendered).subscribe('DIO_SPELLBOX_CHANGE_OPEN', function () {
if(spellbox.show == false) {
spellbox.show = true;
saveValue("spellbox", JSON.stringify(spellbox));
}
changeSpellBox();
});
$.Observer(uw.GameEvents.ui.layout_gods_spells.state_changed).subscribe('DIO_SPELLBOX_CLOSE', function () {
spellbox.show = false;
saveValue("spellbox", JSON.stringify(spellbox));
});
}
function initSpellBox(){
try {
$('').appendTo('head');
$(".gods_spells_menu").css({
width: "134px"
});
$("#ui_box .gods_area .gods_spells_menu .content").css({
background: "url(http://gpzz.innogamescdn.com/images/game/layout/power_tile.png) 1px 4px",
overflow: "auto",
margin: "0 0 0px 0px",
border: "3px inset rgb(16, 87, 19)",
borderRadius: "10px"
});
$('.nui_units_box').css({
display: 'block',
marginTop: '-8px',
position: 'relative'
});
$('.nui_units_box .bottom_ornament').css({
marginTop: '-28px',
position: 'relative'
});
$('.gods_area').css({
height: '150px'
});
if($(".gods_spells_menu .left").get(0)){
$(".gods_spells_menu .left").remove(); $(".gods_spells_menu .right").remove();
$(".gods_spells_menu .top").remove(); $(".gods_spells_menu .bottom").remove();
}
$(".gods_spells_menu").draggable({
containment: "body",
distance: 10 ,
snap: "body, .gods_area, .nui_units_box, .ui_quickbar, .nui_main_menu, .minimized_windows_area, #island_quests_overview",
opacity: 0.7,
stop : function(){
spellbox.top = this.style.top;
spellbox.left = this.style.left;
saveValue("spellbox", JSON.stringify(spellbox));
}
});
$(".gods_area .gods_spells_menu").before($('.nui_units_box'));
// Position
$('.gods_spells_menu').css({
position: 'absolute',
left: spellbox.left,
top: spellbox.top,
zIndex: '5000',
padding: '30px 0px 0px -4px'
});
// Active at game start?
if(spellbox.show) {
$('.btn_gods_spells').click();
}
} catch(error){
errorHandling(error, "initSpellBox");
}
}
function changeSpellBox(){
try {
$(".gods_spells_menu").css({
//height: "300px"
//height: $(".nui_units_box").height() + "px"
});
//console.log($(".nui_units_box").height());
$('.god_container[data-god_id="zeus"]').css({
//float: 'left'
});
$('.powers_container').css({
background: 'none'
});
$('.god_container').css({
float: 'left'
});
$('.god_container[data-god_id="zeus"], .god_container[data-god_id="athena"]').css({
float: 'none'
});
$('.content .title').each(function(){
$(this).get(0).style.display = "none";
});
$('.god_container[data-god_id="poseidon"]').prependTo('.gods_spells_menu .content');
$('.god_container[data-god_id="athena"]').appendTo('.gods_spells_menu .content');
$('.god_container[data-god_id="artemis"]').appendTo('.gods_spells_menu .content');
if($('.bolt').get(0)) { $('.bolt').remove(); }
if($('.earthquake').get(0)) { $('.earthquake').remove(); }
if($('.pest').get(0)) { $('.pest').remove(); }
} catch(error){
errorHandling(error, "changeSpellBox");
}
}
// Minimize Daily reward window on startup
function minimizeDailyReward(){
/*
$.Observer(uw.GameEvents.window.open).subscribe('DIO_WINDOW', function(u,dato){});
$.Observer(uw.GameEvents.window.reload).subscribe('DIO_WINDOW2', function(f){});
*/
if(MutationObserver) {
var startup = new MutationObserver(function(mutations) {
mutations.forEach(function(mutation) {
if(mutation.addedNodes[0]){
if($('.daily_login').get(0)){ // && !uw.GPWindowMgr.getOpenFirst(uw.Layout.wnd.TYPE_SHOW_ON_LOGIN).isMinimized()
$('.daily_login').find(".minimize").click();
//uw.GPWindowMgr.getOpenFirst(uw.Layout.wnd.TYPE_SHOW_ON_LOGIN).minimize();
}
}
});
});
startup.observe($('body').get(0), { attributes: false, childList: true, characterData: false});
setTimeout(function(){ startup.disconnect();}, 3000);
}
}
// Larger taskbar
function scaleTaskbar(){
$('.minimized_windows_area').get(0).style.width= "150%";
$('.minimized_windows_area').get(0).style.left= "-25%";
}
// hide fade out buttons => only for myself
function hideNavElements() {
if(uw.Game.premium_features.curator<=uw.Timestamp.now()){
$('.nav').each(function() {
$(this).get(0).style.display = "none";
});
}
}
/*******************************************************************************************************************************
* Modify Chat
*******************************************************************************************************************************/
function popupChatUser(){ // not used yet
setTimeout(function(){
GM_xmlhttpRequest({
method: "POST",
url: "http://wwwapi.iz-smart.net/modules.php?name=Chaninfo&file=nicks&chan=Grepolis"+ uw.Game.market_id.toUpperCase(),
onload: function(response) {
//$('.nui_main_menu .chat .indicator').get(0).innerHTML =
//console.log(response.responseText);
//$('.nui_main_menu .chat .indicator').get(0).style.display = 'inline';
}
});
}, 0);
}
function initChatUser(){
$('.nui_main_menu .chat .button, .nui_main_menu .chat .name_wrapper').css({
filter: 'url(#Hue1)',
WebkitFilter: 'hue-rotate(65deg)'
});
updateChatUser();
setInterval(function(){ updateChatUser(); }, 300000);
$('.nui_main_menu .chat').mouseover(function(){
//popupChatUser();
});
if($('.nui_main_menu .chat').hasClass('disabled')){ $('.nui_main_menu .chat').removeClass('disabled');}
}
function updateChatUser(){
var market = uw.Game.market_id;
if(gm){
// GM-BROWSER:
chatUserRequest();
} else {
// SAFARI:
$.ajax({
url:"http://diotools.pf-control.de/game/chatuser_count.php?chan=Grepo"+ (market === "de" ? "lisDE" : ""),
dataType : 'text',
success: function(text) {
$('.nui_main_menu .chat .indicator').get(0).innerHTML = text;
$('.nui_main_menu .chat .indicator').get(0).style.display = 'block';
},
error: function (xhr, ajaxOptions, thrownError) {
$('.nui_main_menu .chat .indicator').get(0).style.display = 'none';
}
});
}
}
$('').appendTo('head');
// Modify chat window
function modifyChat() {
var host = { fr: 'irc.quakenet.org', def: 'flash.afterworkchat.de'},
market = uw.Game.market_id, select_nick = false, chatwnd_id,
nickname = uw.Game.player_name;
setTimeout(function(){ updateChatUser(); }, 10000); setTimeout(function(){ updateChatUser(); }, 30000);
//uw.GPWindowMgr.Create(uw.Layout.wnd.TYPE_CHAT);
//uw.GPWindowMgr.getOpenFirst(uw.Layout.wnd.TYPE_CHAT).setWidth(600);
//uw.GPWindowMgr.getOpenFirst(uw.Layout.wnd.TYPE_CHAT).setHeight(300);
//uw.GPWindowMgr.getOpenFirst(uw.Layout.wnd.TYPE_CHAT).setPosition([0,'bottom']);
//console.log(uw.GPWindowMgr.getOpenFirst(uw.Layout.wnd.TYPE_CHAT));
chatwnd_id = '#gpwnd_' + uw.GPWindowMgr.getOpenFirst(uw.Layout.wnd.TYPE_CHAT).getID();
$('#chat').get(0).innerHTML = "";
//$(chatwnd_id).parent().children('.gpwindow_left').remove();
//$(chatwnd_id).parent().children('.gpwindow_right').remove();
//$(chatwnd_id).parent().children('.gpwindow_top').remove();
//$(chatwnd_id).parent().children('.gpwindow_bottom').remove();
//$(chatwnd_id).parent().parent().children('.ui-dialog-titlebar').remove();
var replaceArray = {
// Russian:
"Ё":"YO","Й":"I","Ц":"TS","У":"U","К":"K","Е":"E","Н":"N","Г":"G","Ш":"SH","Щ":"SCH","З":"Z","Х":"H","Ъ":"'",
"ё":"yo","й":"i","ц":"ts","у":"u","к":"k","е":"e","н":"n","г":"g","ш":"sh","щ":"sch","з":"z","х":"h","ъ":"'",
"Ф":"F","Ы":"I","В":"V","А":"a","П":"P","Р":"R","О":"O","Л":"L","Д":"D","Ж":"ZH","Э":"E",
"ф":"f","ы":"i","в":"v","а":"a","п":"p","р":"r","о":"o","л":"l","д":"d","ж":"zh","э":"e",
"Я":"Ya","Ч":"CH","С":"S","М":"M","И":"I","Т":"T","Ь":"'","Б":"B","Ю":"YU",
"я":"ya","ч":"ch","с":"s","м":"m","и":"i","т":"t","ь":"'","б":"b","ю":"yu",
// Greek:
'Α':'A','Β':'B','Γ':'G','Δ':'D','Ε':'E','Ζ':'Z','Η':'H','Θ':'Th','Ι':'I','Κ':'K','Λ':'L','Μ':'M','Ν':'N','Ξ':'J','Ο':'O','Π':'P','Ρ':'R','Σ':'S',
'Τ':'T','Υ':'U','Φ':'F','Χ':'Ch','Ψ':'Ps','Ω':'W','Ά':'A','Έ':'E','Ή':'H','Ί':'I','Ό':'O','Ύ':'U','Ώ':'W','Ϊ':'I',
'α':'a','β':'b','γ':'g','δ':'d','ε':'e','ζ':'z','η':'h','θ':'th','ι':'i','κ':'k','λ':'l','μ':'m','ν':'n','ξ':'j','ο':'o','π':'p','ρ':'r','ς':'s',
'σ':'s','τ':'t','υ':'u','φ':'f','χ':'ch','ψ':'ps','ω':'w','ά':'a','έ':'e','ή':'h','ί':'i','ό':'o','ύ':'u','ώ':'w','ϊ':'i','ΐ':'i'
};
function replaceNick(word){
var temp = "", temp2 = "";
// Step 1: Replace Special and some german chars
word = word.replace(/[.,:,+,*]/g,"").replace(/[=,\ ,\-]/g,"_").replace(/ö/gi,"oe").replace(/ä/gi,"ae").replace(/ü/gi,"ue").replace(/ß/g,"ss");
// Step 2: Replace russian and greek chars
if(!word.match(/^[a-zA-Z0-9_]+$/)){
temp = word.split('').map(function (char) {
var ch = "";
ch = replaceArray[char] || char;
return ch;
}).join("");
// Step 3: Delete all other special chars
if(!temp.match(/^[a-zA-Z0-9_]+$/)){
for(var c = 0; c < temp.length; c++){
if(temp[c].match(/^[a-zA-Z0-9_]+$/)){
temp2 += temp[c];
}
}
select_nick = true;
temp = temp2;
}
word = temp;
}
return word;
}
//nickname = "kνnmδενεί-ναισυνδεδ*εμένος_Ιππέας"; // test nickname
nickname = replaceNick(nickname);
if(PID == 84367){ nickname = "DionY_"; }
$('
').appendTo("#chat");
/*
$('
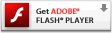
'+
'').appendTo("#chat");
*/
}
/*******************************************************************************************************************************
* Activity boxes
* ----------------------------------------------------------------------------------------------------------------------------
* | ● Show troops and trade activity boxes
* | ● Boxes are magnetic & movable (position memory)
* ----------------------------------------------------------------------------------------------------------------------------
*******************************************************************************************************************************/
var mut_toolbar, mut_command, mut_trade;
function checkToolbarAtStart(){
if(parseInt($('.toolbar_activities .commands .count').get(0).innerHTML, 10) > 0){
$('#toolbar_activity_commands_list').get(0).style.display = "block";
} else {
$('#toolbar_activity_commands_list').get(0).style.display = "none";
}
if(parseInt($('.toolbar_activities .trades .count').get(0).innerHTML, 10) > 0){
$('#toolbar_activity_trades_list').get(0).style.display = "block";
} else {
$('#toolbar_activity_trades_list').get(0).style.display = "none";
}
}
function catchToolbarEvents(){
mut_toolbar = new MutationObserver(function(mutations) {
mutations.forEach(function(mutation) {
if(mutation.addedNodes[0]){
//console.log(mutation);
if(mutation.target.id === "toolbar_activity_trades_list"){
draggableTradeBox();
} else {
draggableCommandBox();
}
mutation.addedNodes[0].remove();
}
});
});
mut_command = new MutationObserver(function(mutations) {
mutations.forEach(function(mutation) {
if(mutation.addedNodes[0]){
if(mutation.addedNodes[0].nodeValue > 0){
$('#toolbar_activity_commands_list').get(0).style.display = "block";
} else {
$('#toolbar_activity_commands_list').get(0).style.display = "none";
}
}
});
});
mut_trade = new MutationObserver(function(mutations) {
mutations.forEach(function(mutation) {
if(mutation.addedNodes[0]){
if(mutation.addedNodes[0].nodeValue > 0){
$('#toolbar_activity_trades_list').get(0).style.display = "block";
} else {
$('#toolbar_activity_trades_list').get(0).style.display = "none";
}
}
});
});
}
// moveable boxes
function draggableTradeBox(){
$("#toolbar_activity_trades_list").draggable({
containment: "body",
distance: 20,
snap: "body, .gods_area, .nui_units_box, .ui_quickbar, .nui_main_menu, .minimized_windows_area, .nui_left_box",
opacity: 0.7,
start : function () {
$("#fix_trade").remove();
},
stop : function () {
var pos = $('#toolbar_activity_trades_list').position();
tradebox.left = pos.left;
saveValue("tradebox", JSON.stringify(tradebox));
$('').appendTo('head');
}
});
}
function draggableCommandBox(){
$("#toolbar_activity_commands_list").draggable({
containment: "body",
distance: 20,
snap: "body, .gods_area, .nui_units_box, .ui_quickbar, .nui_main_menu, .minimized_windows_area, .nui_left_box",
opacity: 0.7,
stop : function () {
var pos = $('#toolbar_activity_commands_list').position();
commandbox.left = pos.left;
commandbox.top = pos.top;
saveValue("commandbox", JSON.stringify(commandbox));
}
});
}
function showActivityBoxes(){
var observe_options = { attributes: false, childList: true, characterData: false};
catchToolbarEvents();
mut_toolbar.observe($('#toolbar_activity_commands_list').get(0), observe_options );
mut_toolbar.observe($('#toolbar_activity_trades_list').get(0), observe_options );
mut_command.observe($('.toolbar_activities .commands .count').get(0), observe_options );
mut_trade.observe($('.toolbar_activities .trades .count').get(0), observe_options );
$('#toolbar_activity_commands').mouseover();
$('#toolbar_activity_trades').mouseover();
$('#toolbar_activity_commands, #toolbar_activity_trades').unbind("mouseover");
$('#toolbar_activity_commands, #toolbar_activity_commands_list, #toolbar_activity_trades, #toolbar_activity_trades_list').unbind("mouseout");
$('#toolbar_activity_trades_list').unbind("click");
checkToolbarAtStart();
$('#toolbar_activity_commands_list').css({
left: commandbox.left + "px",
top: commandbox.top + "px"
});
$(''+
'').appendTo('head');
draggableCommandBox();
draggableTradeBox();
$('.toolbar_activities .commands').mouseover(function(){
$('#toolbar_activity_commands_list').get(0).style.display = "block";
});
$('.toolbar_activities .trades').mouseover(function(){
$('#toolbar_activity_trades_list').get(0).style.display = "block";
});
}
/*******************************************************************************************************************************
* Other stuff
*******************************************************************************************************************************/
function counter(time){
var type = "", today, counted, year, month, day;
if(uw.Game.market_id !== "zz"){
counted = DATA.count;
today = new Date((time + 7200) * 1000);
year = today.getUTCFullYear();
month = ((today.getUTCMonth()+1) < 10 ? "0" : "") + (today.getUTCMonth()+1);
day = (today.getUTCDate() < 10 ? "0" : "") + today.getUTCDate();
today = year + month + day;
//console.log(today);
if(counted[0] !== today){type += "d"; }
if(counted[1] == false){ type += "t"; }
if((counted[2] == undefined) || (counted[2] == false)){ type += "b"; }
if(type !== ""){
$.ajax({
type: "GET",
url: "http://diotools.pf-control.de/game/count.php?type="+ type + "&market="+ uw.Game.market_id + "&date="+ today + "&browser="+ getBrowser(),
dataType : 'text',
success: function (text) {
if(text.indexOf("dly") > -1){
counted[0] = today;
}
if(text.indexOf("tot") > -1){
counted[1] = true;
}
if(text.indexOf("bro") > -1){
counted[2] = true;
}
saveValue("dio_count", JSON.stringify(counted));
}
});
}
}
}
/*
function xmas(){
$('
').appendTo('#ui_box');
$('#xmas').css({
background: 'url("http://www.greensmilies.com/smile/smiley_emoticons_weihnachtsmann_nordpol.gif") no-repeat',
height: '51px',
width: '61px',
position:'absolute',
bottom:'10px',
left:'60px',
zIndex:'2000'
});
$('#xmas').tooltip("HO HO HO, Frohe Weihnachten!");
}
function silvester(){
$('
').appendTo('#ui_box');
$('#silv').css({
//background: 'url("http://www.greensmilies.com/smile/buchstaben_0.gif") no-repeat',
//height: '57px',
//width: '34px',
position:'absolute',
bottom:'10px',
left:'70px',
zIndex:'10'
});
$('#silv').tooltip("Frohes Neues!");
}
function joke(){
setTimeout(function(){
if($('#grcgrc').get(0)){
$('
').appendTo('#ui_box');
$('#fight').css({
background: 'url("http://www.greensmilies.com/smile/smiley_emoticons_hoplit_speer4.gif") no-repeat',
height: '51px',
width: '61px',
position:'absolute',
bottom:'10px',
left:'39px',
zIndex:'2000'
});
$('#fight').tooltip("WWW.GREENSMILIES.COM");
}
}, 5000);
}
*/
}